[Javascript] 배열을 검색해서 원하는 요소를 찾는 방법 총정리
1. indexOf
배열을 검색한다고 하면 90%는 이 함수로 배열에서 원하는 요소의 위치를 찾는 것입니다.
대체로는 단일 데이터 타입으로 된 배열에서 원하는 요소를 빠르게 찾는 단순한 검색에 사용됩니다.
const array=['라이언','어피치','24','콘','프로도',24,'무지',undefined,null,24];
console.log(array.indexOf('콘'));//3
console.log(array.indexOf('24'));//2
console.log(array.indexOf(24));//5
console.log(array.indexOf(undefined));//7
console.log(array.indexOf(null));//8
indexOf() 함수는 몇 가지 간단한 규칙이 있습니다.
- 데이터 타입을 구분하므로 '24', 24는 다른 배열 인덱스를 반환합니다.
- 검색값과 배열 값이 일치하는 배열 요소 중 첫 번째 나오는 배열 인덱스를 반환합니다.(정확히는 처음 일치하는 값이 나오면 인덱스를 반환하고 검색을 멈춥니다.)
- undefined, null과 같은 특별한 데이터 타입 요도 검색할 수 있습니다.
2. findIndex
인자로 함수를 넘겨서 함수에서 검색 필터링/후처리를 해서 원하는 값을 찾을 수 있습니다.
복잡한 검색 조건을 필요로 할 때, 또는 객체 배열에서 개별 객체의 속성이나 값을 검색할 필요가 있을 때 사용할 수 있습니다.
이름(name), 나이(age) 속성 2개를 가진 객체 배열에서 나이가 7보다 큰 첫 번째 배열 요소 인덱스를 반환하는 검색 기능을 다음과 같이 만들 수 있습니다.
const array2=[{name:'라이언', age:5},{name:'어피치', age:3},{name:'콘', age:2},{name:'프로도', age:8}];
console.log(array2.findIndex(checkOld));
function checkOld(arrItem){
return arrItem.age > 7;
}
구분이 쉽도록 별도 함수로 구현해서 함수명을 인자로 전달했지만, 간결하게 다음과 같이 짧게 화살표 함수로 작성하는 것도 괜찮은 방법입니다.
array2.findIndex((arrItem=>arrItem.age>7));
실전에서는 쿼리선택자로 요소들을 선택해서 선택한 요소들 중에서 특정 클래스를 가진 요소를 찾는데도 활용할 수 있습니다.
다음과 같은 HTML에서 클래스 "d"를 가진 첫 번째 요소를 찾아보겠습니다.
<div class="a b c">
<div class="a"></div>
<div class="b"></div>
<div class="c">
<div class="a b"></div>
<div class="a c"></div>
<div class="d"></div>
</div>
<div class="a c d"></div>
</div>
쿼리 선택자로 선택한 요소들을 가진 노드리스트를 펼침 연산자를 활용해 배열로 바꾼 후, 배열의 개별 요소(노드 객체)가 클래스 "d"가 있는지 찾는 함수를 작성해서 findIndex() 함수 인자로 넣으면 됩니다.
const array3 = [...document.querySelectorAll('div')];
console.table(array3);
console.log(array3.findIndex(node=>node.classList.contains('d')));
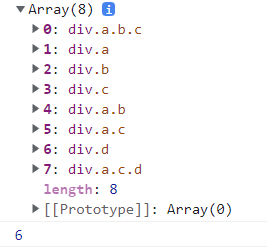
3. includes
배열에 검색 값과 일치하는 요소가 있는지 여부를 알려주는 함수입니다.
반환 값은 불리언이며, true, false 중 하나가 됩니다. 배열이 특정 조건을 만족하는지 체크를 해서 조건 분기 처리를 할 때 유용합니다.
const array4=['2023-01-05','2023-01-07','2023-01-08','2023-01-12','2023-01-15'];
if(array4.includes('2023-01-15')){
console.log('주문 있음.');
}